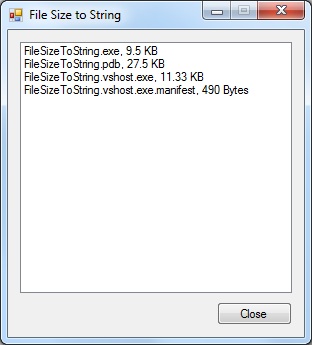
Download Source Code
Introduction
In case you missed it, a bit of strangeness goes on when most software displays the size of a file.
For example, one kilobyte (1 KB) is closely associated with a thousands bytes, but a kilobyte is not exactly the same as a thousand bytes. Similarly, one megabyte (1 MB) is closely associated with a million bytes, but a megabyte is not exactly the same as a million bytes. This doesn't apply only to file sizes. It also applies to measurements of disk capacity, memory or anything else where we're counting bytes.
The convention used to measure bytes was influenced heavily by base-two (binary) numbering. For example, where a million is 1,000 x 1,000, a megabyte is 1,024 x 1,024. This is because 1,024 is exactly a power of two (two to the power of ten), and so this system has been adopted when measuring bytes.
So if you want to display the size of a file in your own application, just calling ToString() on the variable that holds the size of a file in bytes probably won't be sufficient. Instead, you'll probably want to use the same size format used by programs like Windows Explorer.
This format is not only what most users will expect, but it also allows you to shorten the file-size string using suffixes like KB, MB, etc. For example, instead of "1, 048,576 bytes", you could just print "1 MB".
Creating a File-Size-To-String Method
Okay, so what this indicates to me is that it's worth writing a little method that will convert from integer values to file-size strings. Then I can simply use this method any time I want to display a number that represents a count of bytes.
Listing 1 shows my FileSize class. As you can see, it's very simple with one static method, ToString(), which makes the conversion already described.
ToString() takes a long argument that represents the size of a file or other byte count. The method attempts to reduce the value by dividing it by 1,024 as many times as possible. It also tracks the number of times this division occurs and uses that number to determine the correct suffix.
Listing 1: The FileSize Class
class FileSize
{
protected static string[] _suffix = { "Bytes", "KB", "MB", "GB", "TB", "PB", "EB", "ZB", "YB" };
protected const double _fileSizeDivisor = 1024.0;
/// <summary>
/// Converts a file size value to a size string
/// </summary>
/// <param name="sizeinBytes">Size in bytes</param>
/// <returns></returns>
public static string ToString(long sizeInBytes)
{
double size = (double)sizeInBytes;
int suffix = 0;
while (size >= _fileSizeDivisor)
{
suffix++;
size /= _fileSizeDivisor;
}
return String.Format("{0:#,##0.##} {1}", size, _suffix[suffix]);
}
}
Conclusion
And that's about all there is to it. Very simple. But I've ended up using this code quite a bit.
End-User License
Use of this article and any related source code or other files is governed
by the terms and conditions of
.
Author Information
Jonathan Wood
I'm a software/website developer working out of the greater Salt Lake City area in Utah. I've developed many websites including Black Belt Coder, Insider Articles, and others.