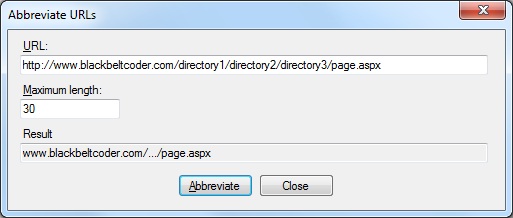
Download Source Code
Introduction
Recently, I had a case where an ASP.NET page displayed the user's website address in a side column. This worked fine except that some users had very long URLs, which were too wide for the column.
Thinking about it, I decided I wanted to simply truncate the visible URL, while still keeping the underlying link intact. However, when I truncated the URL by trimming excess characters, I realized it could be done more intelligently.
For example, consider the URL http://www.domain.com/here/is/one/long/url/page.apsx. If I wanted to keep it within 40 characters, I could trim it to http://www.domain.com/here/is/one/long/u. The problem is that this abbreviation could be more informative. For example, is it a directory or a page? And, if it's a page, what kind? And what exactly does the "u" at the end stand for?
Wouldn't it be a little better if I instead abbreviated this URL to http://www.domain.com/.../url/page.aspx? We've lost a few character places from the three dots used to indicate that information is missing. But we can still see the domain, and the page name and type.
A Smart Function to Abbreviate a URL
The code in Listing 1 abbreviates a URL is this way. The UrlHelper class contains just a single, static method, LimitLength(). This method takes a URL string and maximum length arguments, and attempts to abbreviate the URL so that it will fit within the specified number of characters as described above.
Listing 1: UrlHelper class
public class UrlHelper
{
public static char[] Delimiters = { '/', '\\' };
/// <summary>
/// Attempts to intelligently short the length of a URL. No attempt is
/// made to shorten less than 5 characters.
/// </summary>
/// <param name="url">The URL to be tested</param>
/// <param name="maxLength">The maximum length of the result string</param>
/// <returns></returns>
public static string LimitLength(string url, int maxLength)
{
if (maxLength < 5)
maxLength = 5;
if (url.Length > maxLength)
{
// Remove protocol
int i = url.IndexOfAny(new char[] { ':', '.' });
if (i >= 0 && url[i] == ':')
url = url.Remove(0, i + 1);
// Remove leading delimiters
i = 0;
while (url.Length > 0 && (url[i] == Delimiters[0] || url[0] == Delimiters[1]))
i++;
if (i > 0)
url = url.Remove(0, i);
// Remove trailing delimiter
if (url.Length > maxLength && (url.EndsWith("/") || url.EndsWith("\\")))
url = url.Remove(url.Length - 1);
// Remove path segments until url is short enough or no more segments:
//
// domain.com/abc/def/ghi/jkl.htm
// domain.com/.../def/ghi/jkl.htm
// domain.com/.../ghi/jkl.htm
// domain.com/.../jkl.htm
if (url.Length > maxLength)
{
i = url.IndexOfAny(Delimiters);
if (i >= 0)
{
string first = url.Substring(0, i + 1);
string last = url.Substring(i);
bool trimmed = false;
do
{
i = last.IndexOfAny(Delimiters, 1);
if (i < 0 || i >= (last.Length - 1))
break;
last = last.Substring(i);
trimmed = true;
} while ((first.Length + 3 + last.Length) > maxLength);
if (trimmed)
url = String.Format("{0}...{1}", first, last);
}
}
}
return url;
}
}
If the specified maximum length is less than five, LimitLength() simply changes it to five as there is no point in attempting to shorten a URL to less than the length of the protocol (http://).
Conclusion
That's all there is to it. I hope some of you find this code helpful.
End-User License
Use of this article and any related source code or other files is governed
by the terms and conditions of
.
Author Information
Jonathan Wood
I'm a software/website developer working out of the greater Salt Lake City area in Utah. I've developed many websites including Black Belt Coder, Insider Articles, and others.